Mercury.QTP.CustomServer Namespace : CustomAssistantBase Class |
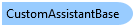
CustomAssistantBase
is the base class for all Record and Replay assistant classes. Assistant class objects are created and run in the AUT context. They help Custom Server objects created in UFT context run parts of their code in the AUT context, thereby gaining direct access to the exposed methods, events, and data of the customized Control. These classes are used only in the UFT context.
Setting up an Assistant Class
Create the Assistant class.
class MyRemoteAssistant : CustomAssistantBase { public int Add(int i, int j) { return(i+j); } }
Create the remote object with CustomServerBase.CreateRemoteObject.
MyRemoteAssistant oMyAssistant = (MyRemoteAssistant)CreateRemoteObject(typeof(MyRemoteAssistant));
Set the target control once before the first use.
oMyAssistant.SetTargetControl(WndHandle.ToInt32());
Invoking Methods of an Assistant Class
Assistant class methods can be invoked directly if they can run in any thread of the AUT.
int i = oMyAssistant.Add(1,2);
Indirect invocation with CustomServerBase.InvokeAssistant causes a method to run in the Control's thread.
int i = (int)InvokeAssistant(oMyAssistant, "Add", 1, 2);
'Declaration
Public Class CustomAssistantBase Inherits System.MarshalByRefObject
public class CustomAssistantBase : System.MarshalByRefObject
System.Object
System.MarshalByRefObject
Mercury.QTP.CustomServer.CustomAssistantBase
Name | Description | |
---|---|---|
![]() | CustomAssistantBase Constructor | Default constructor. |
Name | Description | |
---|---|---|
![]() | TargetControl | Returns the custom control object. |
Name | Description | |
---|---|---|
![]() | GetInprocObjRef | This method supports the UFT infrastructure. Do not override it and do not invoke it directly in your code. |
![]() | InitializeLifetimeService | Overridden. Prevents your Custom Server from timing out. Do not change or override this implementation. |
![]() | SetTargetControl | Attaches to the target custom control object. |