Mercury.QTP.CustomServer Namespace : IEventArgsHelper Interface |
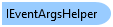
'Declaration
<System.Runtime.InteropServices.ComVisibleAttribute(False)> Public Interface IEventArgsHelper
[System.Runtime.InteropServices.ComVisible(false)] public interface IEventArgsHelper
- MouseEventArgs
- KeyPressEventArgs
- KeyEventArgs
Implementing the IEventArgsHelper
interface includes the following steps:
- Define the Event Arguments helper class or classes.
- Subscribe to events.
Define the Event Arguments helper class or classes
A helper class inherits from the IEventArgsHelper interface and implements its methods.
Note this class must have a default public constructor.
For example, this class serializes MouseEventArgs:[Serializable] public class MyMouseEventArgs : IEventArgsHelper { public MyMouseEventArgs() { } public void Init(EventArgs e) { MouseEventArgs args = (MouseEventArgs)e; Button = args.Button; Clicks = args.Clicks; Delta = args.Delta; X = args.X; Y = args.Y; } public EventArgs GetEventArgs() { MouseEventArgs args; args = new MouseEventArgs(Button, Clicks, Delta, X, Y); return(args); } private MouseButtons Button; private int Clicks; private int Delta; private int X; private int Y; }
Subscribe to events
In the event listening subscription process, use method CustomServerBase.AddHandler, where the third argument is your Event Arguments Helper class type. This tells UFT to serialize the Event Argument of the subscribed event using an instance of Event Arguments Helper class type.
For example, this is a subscription to the MouseUp event:EventHandler e = new MouseEventHandler(this.OnMouseUp); AddHandler("MouseUp", e, typeof(MyMouseEventArgs));
Name | Description | |
---|---|---|
![]() | GetEventArgs | Retrieves and deserializes the EventArgs object. |
![]() | Init | Initializes the Event Arguments helper class with an EventArgs object. |